CombatTarget Lock Ability
Last modified: 14 November 2024Selects a target using the Gameplay Targeting System.
Tracks the selected target's Death Delegate so it can deselect the target automatically.
Can optionally select a new target, after the current one is destroyed.
The Target Lock Ability is part of the Target Lock System. It is responsible for collecting targets, using the Gameplay Targeting System and registering them in the Target Manager.
Since a target lock can happen at any time, whenever a key is pressed or an event is received, this ability should be granted to characters by default.
Targeting Presents
The Target Lock Ability supports two Targeting Presets:
Targeting Preset: Main targeting preset used when the ability activates.
Next Targeting Preset: An optional present used, when Move to Next Targets is enabled. If set to null, the main preset is used.
Distance Tracking
You can optionally track the distance between the avatar and the current target. When tracked, a threshold is established and if the distance is bigger than that value, the target will be unlocked.
Event Activation
Normally, a player would activate the Target Lock Ability by pressing a certain key or button. In these cases you can activate the ability normally, via its Gameplay Tags or Ability Class.
But there might be some situations where the Target Lock Ability should be activated as a consequence of another gameplay circumstance or event. For example:
Automated targeting, where an incoming attacker is automatically targeted.
AI integration, where the perception system automatically locks on the perceived actor.
For those scenarios, the Target Lock Ability can be activated via a Gameplay Event. To that end, this ability will always listen to Gameplay Events represented by these gameplay tags:
Gameplay Tag | Description |
---|---|
| Requests the ability to lock on a target in the payload. |
| Requests the ability to unlock from the current target. |
tip
Ninja Bot Integration
Ninja Bot can automatically send the Gameplay Event to lock and unlock targets obtained via the Perception System.
Target Acquired Gameplay Event
To lock on a pre-determined, you can send the appropriate Gameplay Event, providing the actor in the payload.
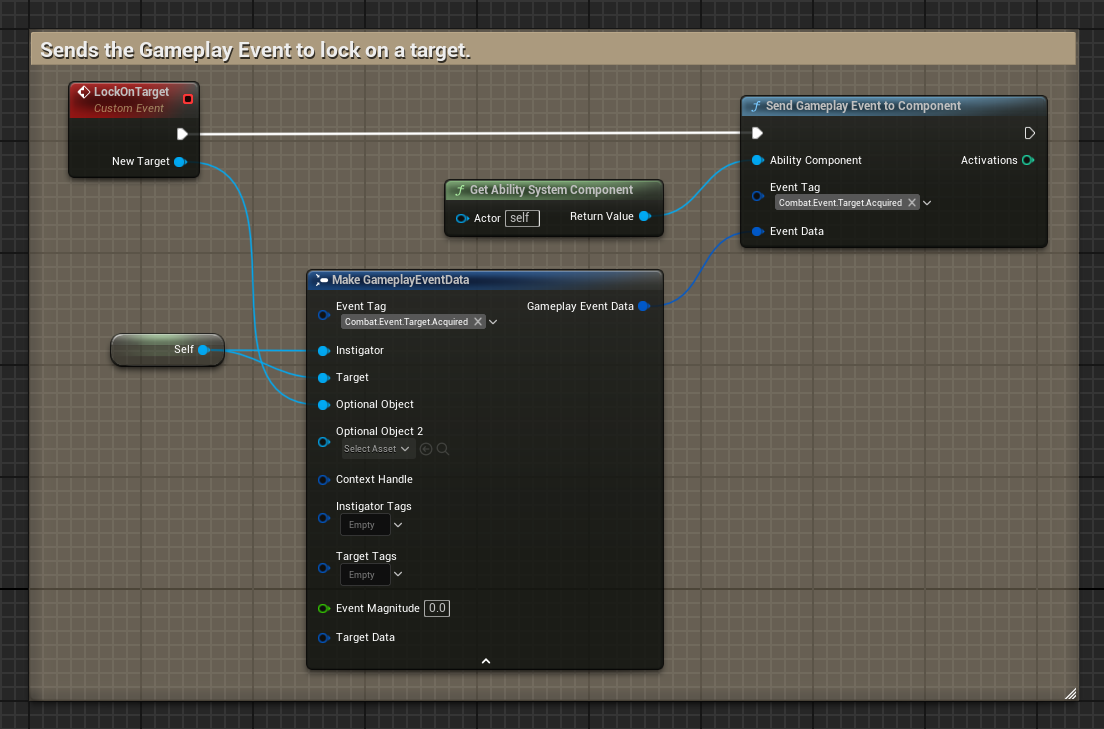
void APluginLabsCharacter::LockOnTarget(AActor* NewTarget)
{
// The Native Gameplay Tag is provided by "NinjaCombatTags.h".
const FGameplayTag EventTag = Tag_Combat_Event_Target_Acquired;
UAbilitySystemComponent* AbilityComponent = UAbilitySystemGlobals::GetAbilitySystemComponentFromActor(this);
if (IsValid(AbilityComponent))
{
FGameplayEventData Payload;
Payload.EventTag = EventTag;
Payload.Instigator = this;
Payload.Target = this;
Payload.OptionalObject = NewTarget;
AbilityComponent->HandleGameplayEvent(EventTag, &Payload);
}
}
Target Dismissed Gameplay Event
To dismiss the current target, you can send the appropriate Gameplay Event, without any additional actors.
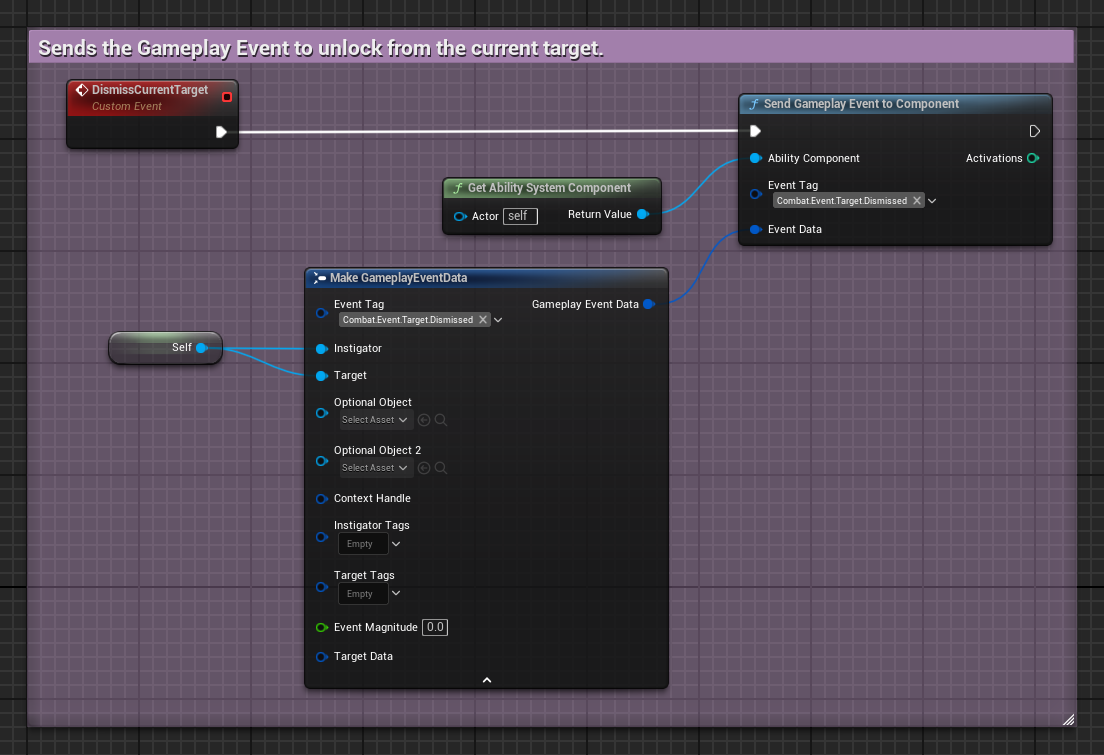
void APluginLabsCharacter::DismissCurrentTarget()
{
// The Native Gameplay Tag is provided by "NinjaCombatTags.h".
const FGameplayTag EventTag = Tag_Combat_Event_Target_Dismissed;
UAbilitySystemComponent* AbilityComponent = UAbilitySystemGlobals::GetAbilitySystemComponentFromActor(this);
if (IsValid(AbilityComponent))
{
FGameplayEventData Payload;
Payload.EventTag = EventTag;
Payload.Instigator = this;
Payload.Target = this;
AbilityComponent->HandleGameplayEvent(EventTag, &Payload);
}
}