Setup
Last modified: 25 March 2025Download and install the plugin.
Ensure that all pre-requisites are completed before configuring the Combat System.
If your project uses C++ make sure to add the correct modules to your `Build.cs` file.
Configure the Ability System elements used by the Combat System.
Add the necessary components and interfaces to your character.
This page will walk you through the pre-requisites and steps necessary to enable the Ninja Combat plugin.
Pre-Requisites
Before setting up the component, there are some pre-requisites to address.
First, ensure you have a working Gameplay Ability System setup:
Add the Ability System Component to your Pawn or Player State.
Properly implement the Ability System Interface on your base class.
Ensure that you can add Attribute Sets, Gameplay Effects, and Gameplay Abilities.
If you are using Ninja G.A.S., configure it first.
Next, make sure you have the input configured:
Your Player Character is ready to handle Input Actions.
If you are using Ninja Input, configure it first.
Installing the Plugin
Once acquired, you can install the plugin via the Epic Games Launcher. Like any code plugin, it can be installed to any compatible engine version.
Once installed, create or open your project and navigate to Edit and then Plugins. In the search bar, type Combat and the Ninja Combat plugin should appear. Tick the checkbox and restart the engine.
C++ Modules
If you're working with C++ and using classes from the Combat System, ensure you add the following modules to your Build.cs
file:
PublicDependencyModuleNames.AddRange(new string[]
{
"CommonUI",
"GameplayAbilities",
"GameplayTags",
"GameplayTasks",
"InputCore",
"ModelViewViewModel",
"Niagara",
"NinjaCombat",
"NinjaCombatCamera",
"NinjaCombatCore",
"NinjaCombatActorPool",
"NinjaCombatUI",
"StructUtils",
"UMG"
});
Ability System
There are two steps to execute during the initial setup: Configure the Ability System Globals and adding the Combat Attribute Set.
As you explore other pages, or the how-tos, you will learn about other GAS-related aspects that can be configured later on.
Ability System Globals
The Ability System must be configured to use the Global Class and the Cue Manager provided by the project, or a custom subclass exclusive to your project.
Add the following settings to your DefaultGame.ini
, located in the Config
folder.
[/Script/GameplayAbilities.AbilitySystemGlobals]
AbilitySystemGlobalsClassName="/Script/NinjaCombat.NinjaCombatAbilitySystemGlobals"
GlobalGameplayCueManagerClass="/Script/NinjaCombat.NinjaCombatGameplayCueManager"
Combat Attribute Set
Add the NinjaCombatAttributeSet
to your character's Ability System Component. You find the sample data to initialize it in the appropriate Attribute Set reference page.
Collision Channels
You may want to create dedicated trace channels for Melee Scans and Projectiles. One way of doing that, for example is by adding the following lines to your DefaultEngine.ini
file, located in your Config
folder.
[/Script/Engine.CollisionProfile]
+DefaultChannelResponses=(Channel=ECC_GameTraceChannel1,DefaultResponse=ECR_Ignore,bTraceType=True,bStaticObject=False,Name="Weapon")
+DefaultChannelResponses=(Channel=ECC_GameTraceChannel2,DefaultResponse=ECR_Block,bTraceType=True,bStaticObject=True,Name="Projectile")
Here is a breakdown of these collision types, so you can make sure that actors will also react correctly to them:
The Weapon channel is used to trace weapon collisions during Melee Attacks.
The Projectile channel is used to detect impacts from Projectile Impacts.
note
Ignore or Block?
You should consider if you want your melee and ranged attacks only interacting with possible targets, or with everything else too, so you could do things like adding decals to walls after hitting them with a sword or fireball.
warning
Double-check your Game Trace Channels
The Trace Channels from the sample above might already be in use for other purposes in your game. Double-check that they are not conflicting with anything else to avoid odd collision issues.
Also make sure that you are not duplicating the Collision Profile category itself.
Finally, assign these Collision Channels in your Combat Settings: Edit
> Project Settings
> Ninja Combat
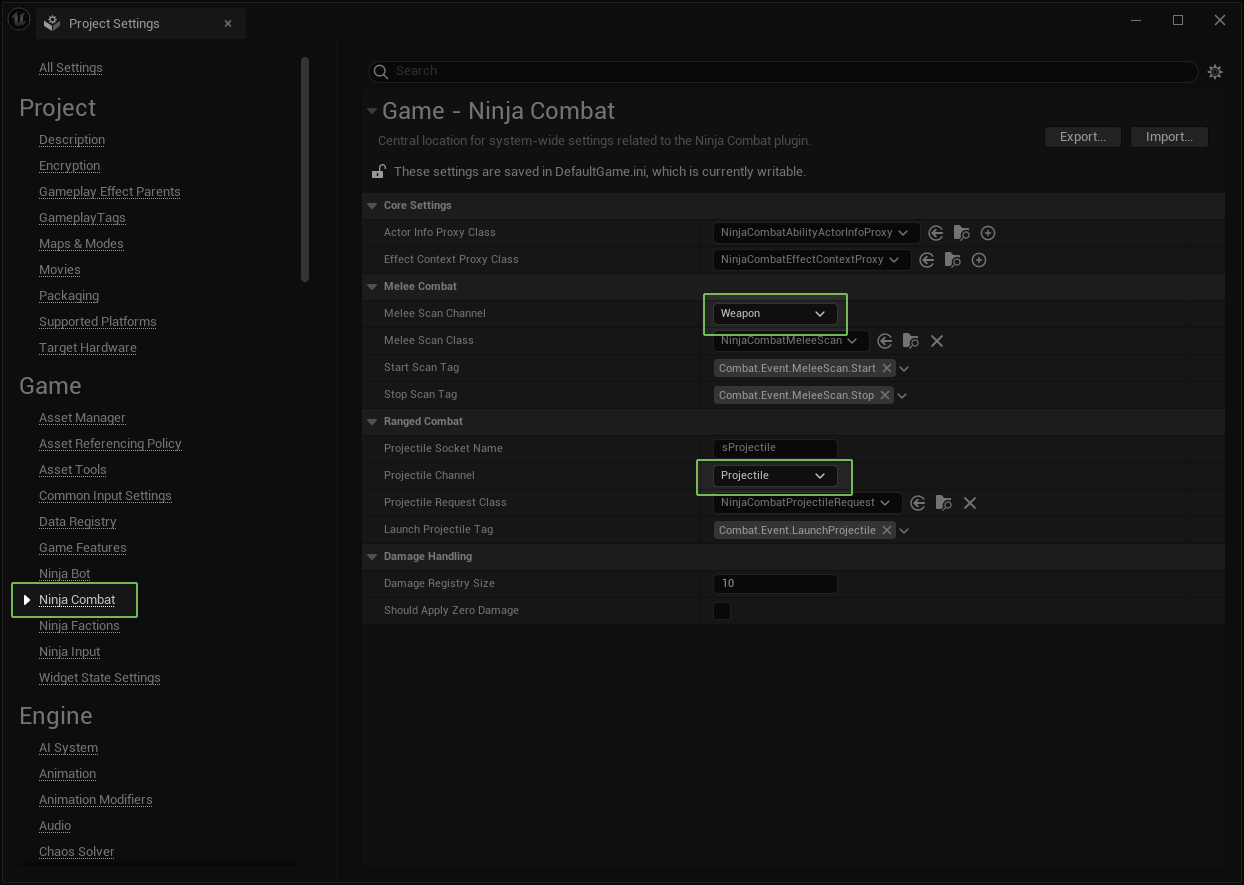
Combat Component
Your main Character class must have the Combat Manager component added to it, along with the appropriate Combat System Interface. Make sure to return the Combat Manager from the GetCombatManager
function.
The Combat Component also must access the Combat Mesh and the proper Animation Blueprint that should be used for Combat Animations. Sometimes, these might be the same, but it is not uncommon for them to be different.
In your base character, implement GetCombatMesh
providing the main mesh for your combatant and if you are using a
note
How about the other Components and Functions?
You don't need to worry about other components or methods in this interface for now, except for the Weapon Manager and Movement Component, both listed below.
For more information about the other components, and how to use these interface functions, check the Extensions page.
Weapon Manager
Create the appropriate Weapon Manager and make sure to return it in the Combat System Interface. By default, you have two options available for the Weapon Manager type:
Default: A basic Weapon Manager that can be used as-is for simpler scenarios.
Equipment: This Weapon Manager is compatible with the Inventory System.
tip
One step at a time
Even if you plan to integrate with Ninja Inventory, consider starting with the Default Weapon Manager, so you can make sure that your system works as expected, before introducing more layers to it.
Movement Component
If you want to use the provided Combat Character Movement Component, make sure to override the default one in your character, either in C++ or in your Blueprint.
You are not required to use this Movement Component in your project, and it is also fine to use a completely different Movement Solution.
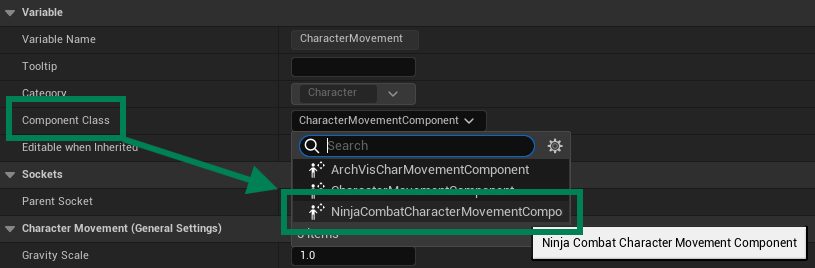
APluginLabsCharacter::APluginLabsCharacter(const FObjectInitializer& ObjectInitializer)
: Super(ObjectInitializer.SetDefaultSubobjectClass<UNinjaCombatCharacterMovementComponent>(CharacterMovementComponentName))
{
// ...
}
Framework Upgrades
warning
Back up first!
Before migrating to a newer version, it is highly recommended that you back up your project first!
1.0 to 2.0
Version 2.0 introduced many updates to the Combat System. It also drastically simplified the configuration. It’s easier to have a separate copy for the migration, so you can paste some contents over.
The combat components "Damage", "Defense" and "Target" were merged into the unified
NinjaCombatManagerComponent
. To get started with the new system, you only need to add that component and aNinjaCombatWeaponManagerComponent
.The
CombatSystemInterface
will try to find all components by their interfaces. Right away, you can just implement the getters for the Combat Manager and Weapon Manager. Other functions can be implemented if/when necessary.The Weapon Manager was improved to be usable out-of-the-box. Review extensions that you made to this component, as they may no longer be needed.
The Opportunity Manager and all related components were removed from the system. Please check the new Opportunity System to learn how to configure Opportunity Attacks.
Weapon Actors have a default Scene Component, and already implement Melee and Projectile interfaces. They have many properties available to adjust their behaviors.
Functions for Weapon Cosmetics, from Melee and Ranged interfaces, had their signature changed. If you have custom implementations for these interfaces, review them accordingly.
In a C++ project, make sure to review your modules in the
Build.cs
file.The death flow was reviewed to be more uniform across different sources of fatal damage. If you made changes to the original functions, you might need to review them.
The Poise and Stagger system was changed and Poise now decreases. This addresses an issue where characters would not react to damage in version 1.0 since their poise was always zero. Be sure to review your Combat Attributes using the new JSON reference.
The Dissolve Handler is more flexible, supporting multiple parameters and curves.
The Forward Reference can be any Scene Component, and it can be created automatically by the Combat Manager.
The Movement Manager has been converted into an actual Character Movement Component, so you will need to add that to your character, if you want to use the movement capabilities.
2.0 to 3.0
Version 3.0 updated some delegates, to make them more compatible with stateless classes such as AI nodes. Your callbacks (C++ and Blueprints) should be reviewed now that most delegates will have an extra value: the component that is broadcasting the event.