Input Setup
Input Setups are Data Assets used to bundle an Input Mapping Context to all appropriate Input Handlers. These bundles are provided to the Input Manager either as default setups or managed during runtime.
You can categorize Input Setups as:
Default: Always available to the player pawn.
Situational: Added or removed based on specific contexts.
Default Input Setups
Default Input Setups are always active and are assigned within the Input Manager Component’s Default Settings.
The system supports multiple Default Input Setups, providing the flexibility to organize your inputs into as many logical bundles as needed.
The specifics of which default setups to add will vary depending on the project's scope. Smaller games might benefit from having all setups added as default. In contrast, larger games may require more deliberate management of input contexts.
Situational Input Setups
Situational Input Setups are activated on-demand and are triggered by specific events, allowing for the addition or removal of Input Setups as needed.
Add and Remove Functions
The Input Manager Component provides dedicated functions to add or remove Input Setups whenever necessary. It’s important to ensure that any context you add is properly removed later to avoid unexpected behavior.
These functions are available in both Blueprints and C++ and can be used as needed. Here are common usage cases:
Begin or End Play of certain actors that provide additional input options to players.
When the User Interface activates and new inputs are available.
Inputs granted, based on certain conditions that must be met.
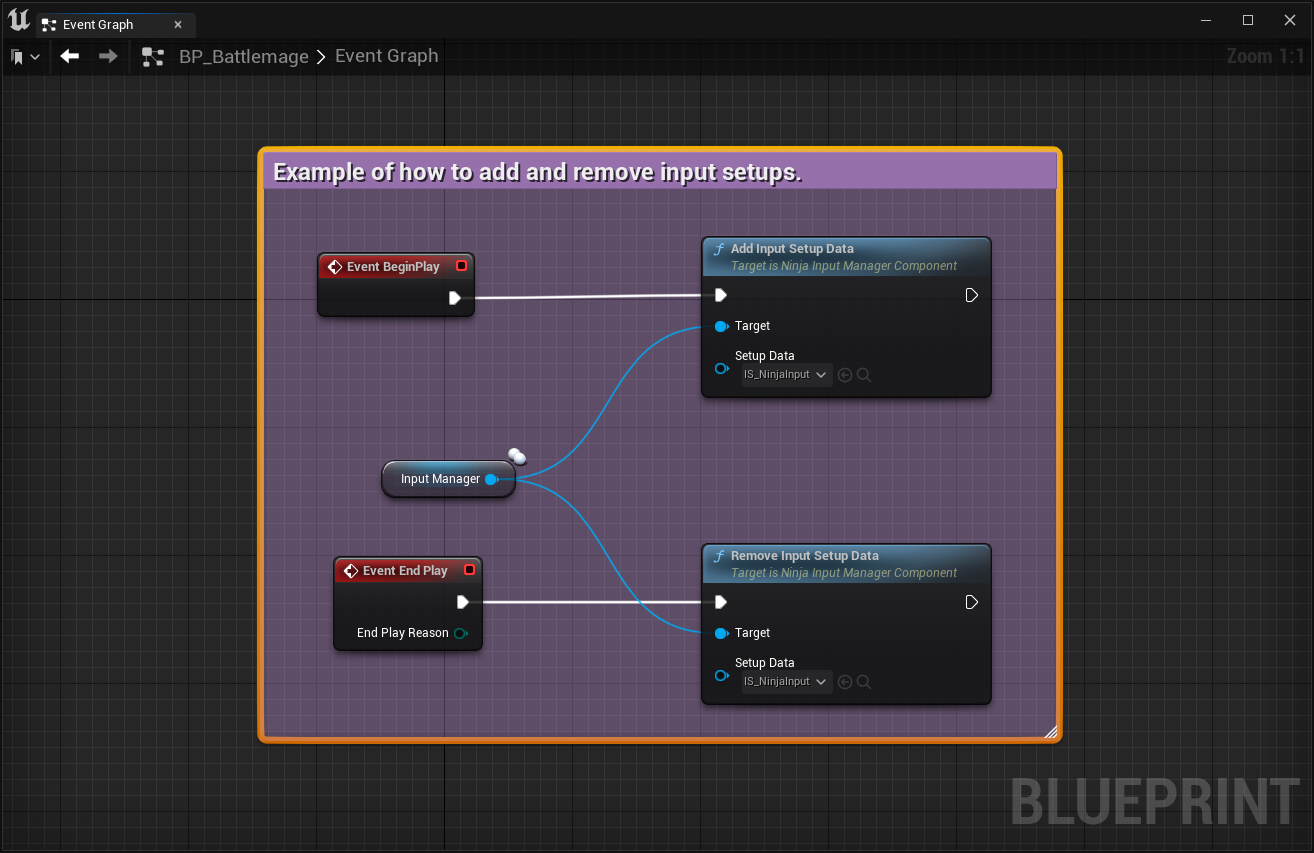
Input Setup Provider Interface
Situational Input Setups can also be managed via the Input Setup Provider Interface.
This interface can be implemented by any pawn. When a Player Controller possesses such a pawn, its Input Manager Component checks for this interface and automatically adds the provided setups. The setups are then removed when the pawn is unpossessed.
Globally Enable or Disable Inputs
Inputs can be completely disabled, and later on enabled at the Input Manager component level.
This can be useful when doing in-game cutscenes or other similar operations where all inputs should be discarded, without the need to remove/add current Setups.