FactionsSetup
Last modified: 15 September 2024Download and install the plugin.
If your project uses C++ make sure to add the correct modules to your `Build.cs` file.
Add the Faction Data Asset to the Asset Manager.
Add the necessary components and interfaces to your character and player controllers.
This page will walk you through the pre-requisites and steps necessary to enable the Ninja Factions plugin.
Installing the Plugin
Once acquired, you can install the plugin via the Epic Games Launcher. Like any code plugin, it can be installed to any compatible engine version.
Once installed, create or open your project and navigate to Edit and then Plugins. In the search bar, type Factions and the Ninja Factions plugin should appear. Tick the checkbox and restart the engine.
C++ Modules
If you're working with C++ and using classes from the Factions System, ensure you add the following modules to your Build.cs
file:
PublicDependencyModuleNames.AddRange(new string[]
{
"AIModule",
"GameplayTags",
"NinjaFactions",
});
Asset Manager
Add the Faction Data Asset to the Asset Manager, ensuring that the correct name and folder are added.
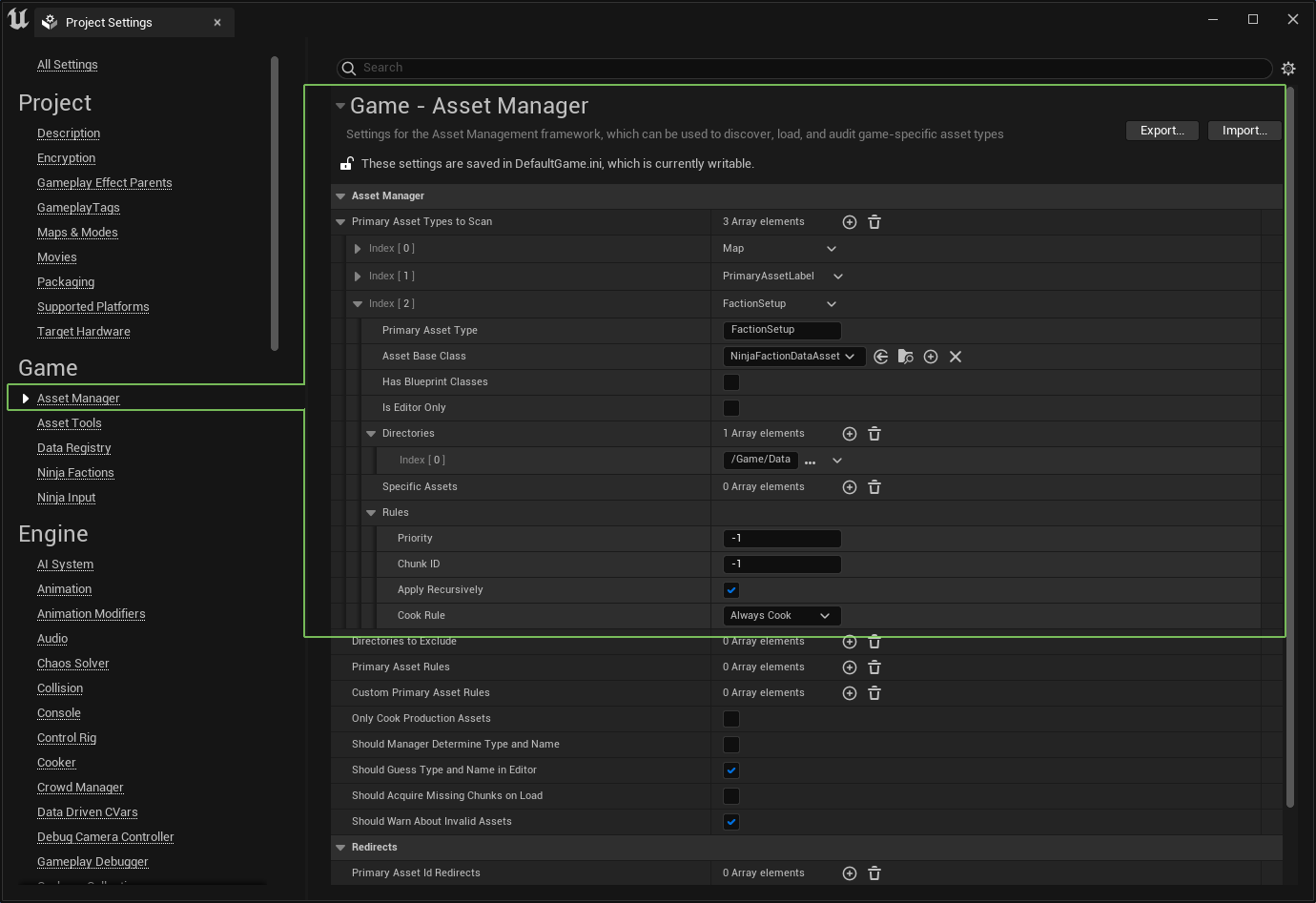
Here's a summary of changes in the new entry:
Setting | Description |
---|---|
Primary Asset Type | Unique name used to identify this asset. The name defined in the class is |
Asset Base Class | Base class that represents this these assets. In our case, |
Directories | All content folders where assets of this type can be found. Adjust this as necessary. |
Cook Rule | Always cook. |
Faction Component
The Faction Component must be present on each actor that belongs to a Faction. The most common examples are certainly the Player or AI characters, but other actors such as vehicles and buildings can have factions too.
Optionally, each Faction Member can implement the Faction Manager Interface (FactionMemberInterface
). This allows the system to quickly retrieve the Faction Manager, without the need to iterate over actor components.
#pragma once
#include "CoreMinimal.h"
#include "GameFramework/Character.h"
#include "Interfaces/FactionMemberInterface.h"
#include "PluginLabsPlayerState.generated.h"
class UNinjaFactionComponent;
UCLASS()
class PLUGINLABS_API APluginLabsCharacter : public ACharacter, public IFactionMemberInterface,
{
GENERATED_BODY()
public:
APluginLabsCharacter(const FObjectInitializer& ObjectInitializer = FObjectInitializer::Get());
// -- Begin Faction Member implementation
virtual UNinjaFactionComponent* GetFactionComponent_Implementation() const override;
// -- End Faction Member implementation
private:
UPROPERTY(EditDefaultsOnly, BlueprintReadOnly, Category = "Components", meta = (AllowPrivateAccess = true))
TObjectPtr<UNinjaFactionComponent> FactionManager;
};
#include "GameFramework/PluginLabsCharacter.h"
#include "Components/NinjaFactionComponent.h"
APluginLabsCharacter::APluginLabsCharacter(const FObjectInitializer& ObjectInitializer) : Super(ObjectInitializer)
{
static const FName FactionComponentName = TEXT("FactionManager");
FactionManager = CreateOptionalDefaultSubobject<UNinjaFactionComponent>(FactionComponentName);
}
UNinjaFactionComponent* APluginLabsCharacter::GetFactionComponent_Implementation() const
{
return FactionManager;
}
Controllers
If you are planning to use Factions to determine the AI Attitude between AI Agents and actors detected by their Perception, then you also need to configure both the AI and Player Controllers.
The Unreal Engine's Perception System will use the GenericTeamAgentInterface
, implemented by Controllers, to determine the attitude between members of certain teams.
Factions are designed to provide a predefined Team ID, and if you are also using Reputation, it can be used to define the attitude.
AI Controller
The AI Controller already implements GenericTeamAgentInterface
, so all that is left is to extend the implementation, so it uses the Faction Manager for attitude checks.
The Faction System provides a base AI Controller, ANinjaFactionAIController
, that is able to retrieve the Faction Manager from its possessed Pawn and, by using the system's Attitude Provider, determine the correct attitude between the agent and its sensed targets.
tip
This AI Controller is optional
If you already have your own hierarchy for the AI Controller, using the one provided by the framework is optional. But you need to make sure that the
GetTeamAttitudeTowards
function uses the Faction Manager.
Player Controller
The Player Controller does not implement the GenericTeamAgentInterface
used by the AI System to determine the attitude between AI and Player Controllers. Furthermore, this interface is not implementable in Blueprints.
The Faction System provides a base Player Controller, NinjaFactionPlayerController
, that is able to retrieve the Faction Manager from its possessed Pawn, and use it to determine the Team ID for the AI System.
tip
This Player Controller is optional
If you already have your own hierarchy for the Player Controller, using the one provided by the framework is optional. But you need to make sure that the interface is correctly implemented, retrieving the Team from the Faction Manager.